Meta Description:
Learn best practices for custom WordPress development with Laravel. Explore how to integrate WordPress and Laravel for headless WordPress solutions and advanced development.
Introduction
In the ever-evolving world of web development, businesses often require highly customized and robust solutions that extend beyond the capabilities of traditional content management systems. Custom WordPress development with Laravel is emerging as a powerful approach to harness the flexibility of WordPress and the elegance of Laravel’s modern PHP framework. By integrating these two technologies, developers can build headless WordPress applications that offer enhanced performance, greater scalability, and a more tailored user experience. In this guide, we’ll explore the best practices for custom WordPress development with Laravel, covering integration techniques, benefits, and real-world examples to help you create smart, efficient web applications.
1. Why Integrate WordPress with Laravel?
1.1 The Need for Customization and Flexibility
Traditional WordPress themes and plugins sometimes limit customization. By combining WordPress with Laravel, you can:
- Achieve Greater Flexibility: Customize both the frontend and backend to meet specific business needs.
- Enhance Performance: Utilize Laravel’s modern features to optimize processes that WordPress alone might struggle with.
- Build Headless Applications: Decouple the frontend from the CMS to deliver faster and more dynamic user experiences.
1.2 Key Benefits of Integration
- Seamless Content Management: WordPress remains a powerful tool for managing content while Laravel handles complex business logic.
- Improved Security and Scalability: Laravel’s robust architecture can enhance security measures and support scalability as your website grows.
- Enhanced Developer Experience: Leverage Laravel’s elegant syntax and powerful features alongside WordPress’s vast ecosystem.
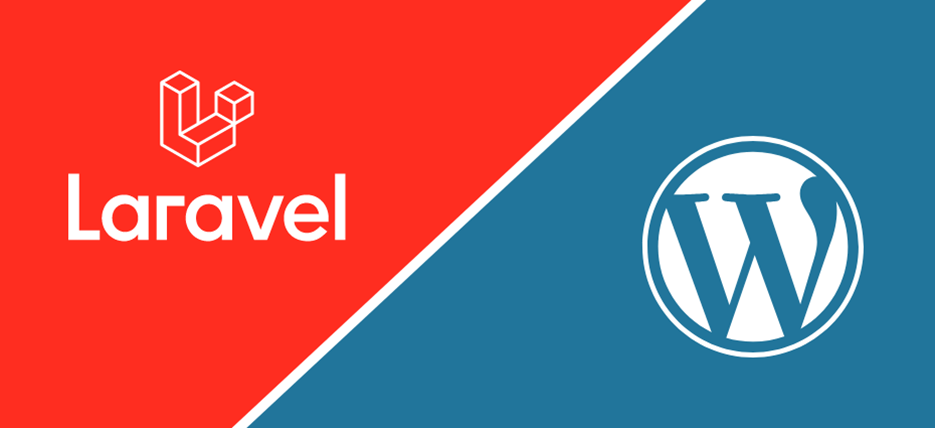
2. Best Practices for Custom WordPress Development with Laravel
2.1 Planning and Strategy
Before diving into development, clearly define your project goals:
- Define Requirements: Identify which parts of your application require custom features beyond standard WordPress capabilities.
- Choose an Architecture: Decide whether to build a headless WordPress solution or integrate Laravel directly with WordPress’s backend.
- Set Milestones: Create a roadmap that outlines development phases, testing, and deployment.
2.2 Maintain a Decoupled Architecture
One of the most effective approaches is to adopt a decoupled, or headless, architecture:
- Backend (WordPress): Use WordPress for content management and data storage.
- Frontend (Laravel): Use Laravel to build a custom frontend that fetches content from WordPress via REST APIs.
This separation allows for:
- Faster Performance: Custom frontend rendering with Laravel’s blade templating engine.
- Improved Flexibility: Ability to scale and update the UI independently from the CMS.
2.3 Use REST APIs for Communication
Leverage WordPress REST APIs to connect your Laravel application with WordPress:
- Fetch Data: Retrieve posts, pages, and other content dynamically.
- Update Content: Allow Laravel to send data back to WordPress when needed.
Code Snippet Example:
// Example: Fetching WordPress posts using Laravel’s HTTP client
use Illuminate\Support\Facades\Http;
$response = Http::get(‘https://yourwordpresssite.com/wp-json/wp/v2/posts’);
$posts = $response->json();
// Display the title of each post
foreach ($posts as $post) {
echo $post[‘title’][‘rendered’] . “\n”;
}
Explanation:
This snippet demonstrates how Laravel’s HTTP client can be used to interact with the WordPress REST API, fetching posts and iterating through them. It’s a simple yet powerful example of decoupled integration.
2.4 Ensure Consistent Data Models
Maintain consistent data models across both platforms:
- Data Mapping: Use Laravel models to represent WordPress data structures.
- Validation: Validate data received from WordPress to prevent errors and security issues.
This approach minimizes data inconsistency and improves overall system reliability.
3. Implementing Headless WordPress with Laravel
3.1 What is Headless WordPress?
Headless WordPress separates the backend (content management) from the frontend (presentation layer). This allows:
- Custom Frontend Development: Build a modern, dynamic UI with Laravel.
- Enhanced Performance: Faster load times and smoother interactions by leveraging Laravel’s capabilities.
3.2 Steps to Build a Headless Setup
- Set Up WordPress:
Install and configure WordPress as your content management system. Enable the REST API (usually enabled by default). - Develop the Laravel Frontend:
Create a new Laravel project to serve as your frontend. Use Laravel’s routing and Blade templating to build your UI. - Connect via API:
Use HTTP clients in Laravel to fetch data from WordPress, as shown in the code snippet above. - Optimize and Test:
Ensure the integration is efficient and secure. Perform load testing to optimize performance.
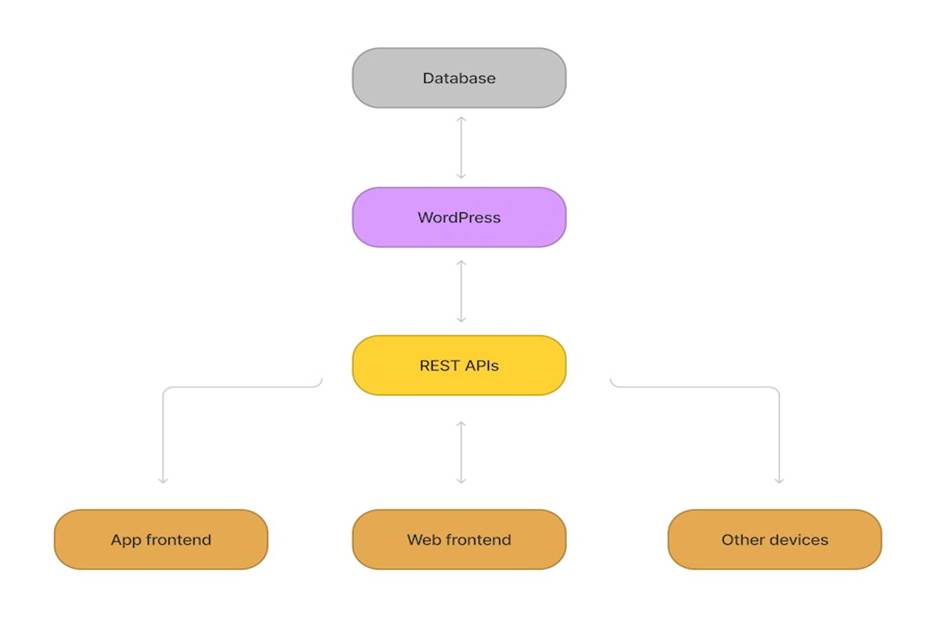
4. Security and Performance Considerations
4.1 Security Best Practices
- Authentication: Secure API endpoints with proper authentication methods (OAuth or JWT).
- Data Sanitization: Sanitize and validate all data exchanged between WordPress and Laravel.
- Regular Updates: Keep both WordPress and Laravel updated to mitigate security vulnerabilities.
4.2 Performance Optimization
- Caching: Use caching mechanisms in Laravel to store frequently accessed data from WordPress.
- Load Balancing: Consider load balancing for high-traffic sites to distribute requests evenly.
- Monitoring: Implement monitoring tools to track performance and quickly identify issues.
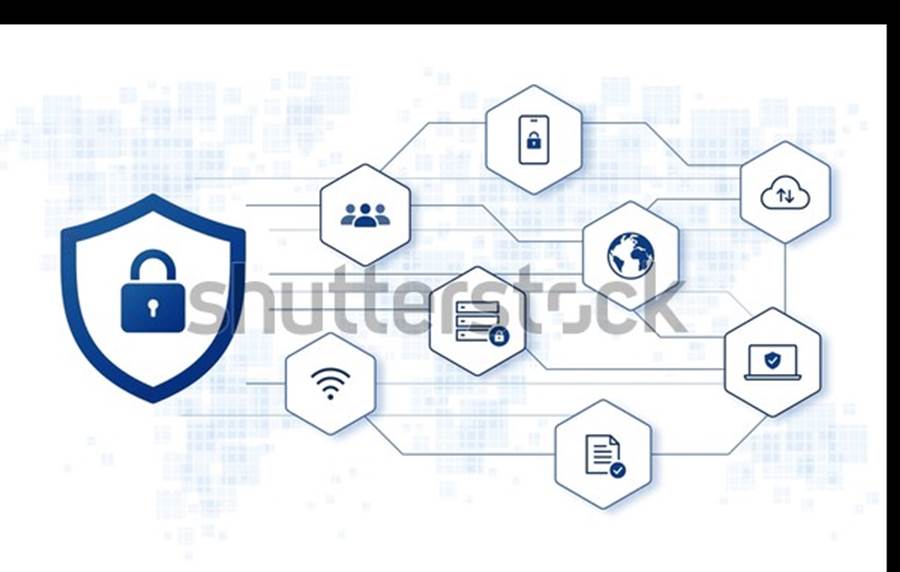
5. Real-world use Cases and Success Stories
5.1 E-Commerce Platforms
E-commerce sites using WordPress for content and product management can benefit from a custom Laravel frontend to deliver a seamless shopping experience.
- Example: An online store that uses WordPress for its CMS and Laravel for a dynamic product catalog and checkout process.
5.2 Enterprise Blogs and Portals
Large enterprises often require highly customized user experiences. By integrating Laravel, companies can build personalized content portals that scale efficiently.
- Example: A corporate blog that uses WordPress for content management but leverages Laravel for advanced user interactions and data visualization.
5.3 Custom Business Solutions
Businesses that need tailor-made applications—such as internal dashboards, customer support systems, or interactive portals—can combine the ease of WordPress with Laravel’s custom development to meet unique requirements.
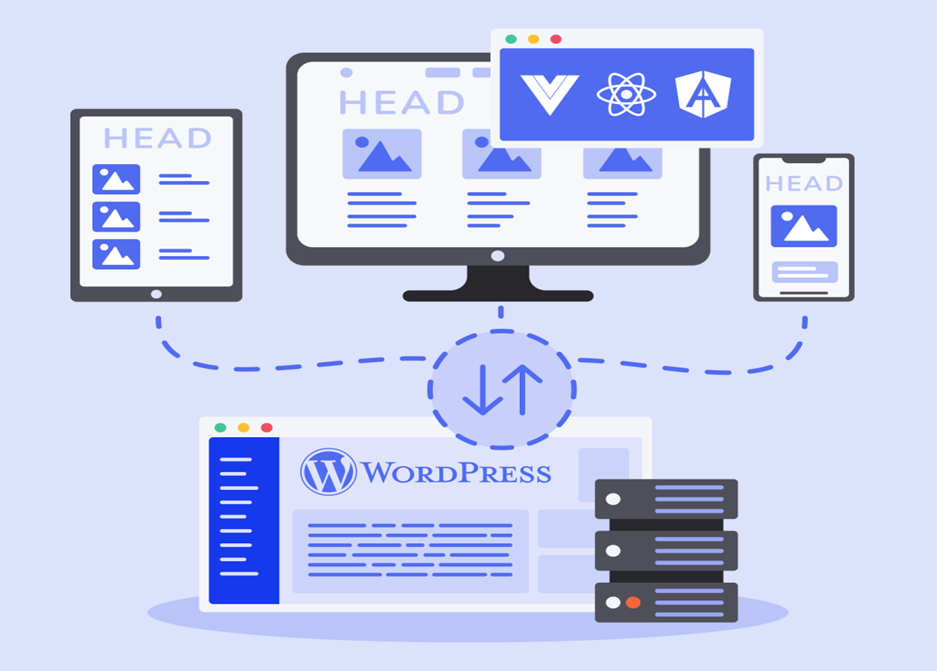
6. Future Trends and Conclusion
6.1 The Future of Custom Web Development
As digital experiences become increasingly complex, the trend toward decoupled architectures will continue to grow. Innovations in AI, progressive web apps (PWAs), and real-time data processing will further enhance the capabilities of custom WordPress development with Laravel.
- Companion Technologies: The integration of tools like GPT can further personalize user interactions and streamline content generation.
6.2 Final Thoughts
Integrating WordPress with Laravel offers a robust solution for modern web development. Whether you choose a headless architecture or a more traditional integration, following best practices in security, performance, and data management is crucial.
By leveraging the strengths of both platforms, businesses can create fast, secure, and engaging web applications that stand out in a competitive digital landscape.
Start your journey towards smarter, custom web development with WordPress and Laravel, and future-proof your digital strategy today!
Visual Summary
- Flutter & AI Integration Graphic:
A side-by-side graphic with WordPress and Laravel logos, highlighting their integration.
(Introduction / Section 1) - Headless Architecture Flowchart:
A flowchart diagram showing the decoupled setup: WordPress (backend) → REST API → Laravel (frontend).
(Section 3) - Security and Performance Infographic:
A visual representation of security measures and performance optimization techniques.
(Section 4) - Case Studies Collage:
An image collage showcasing real-world use cases in e-commerce, enterprise portals, and custom business solutions.
(Section 5)